GPT-4 App Development Guide: Transform App Development with AI
Updated on
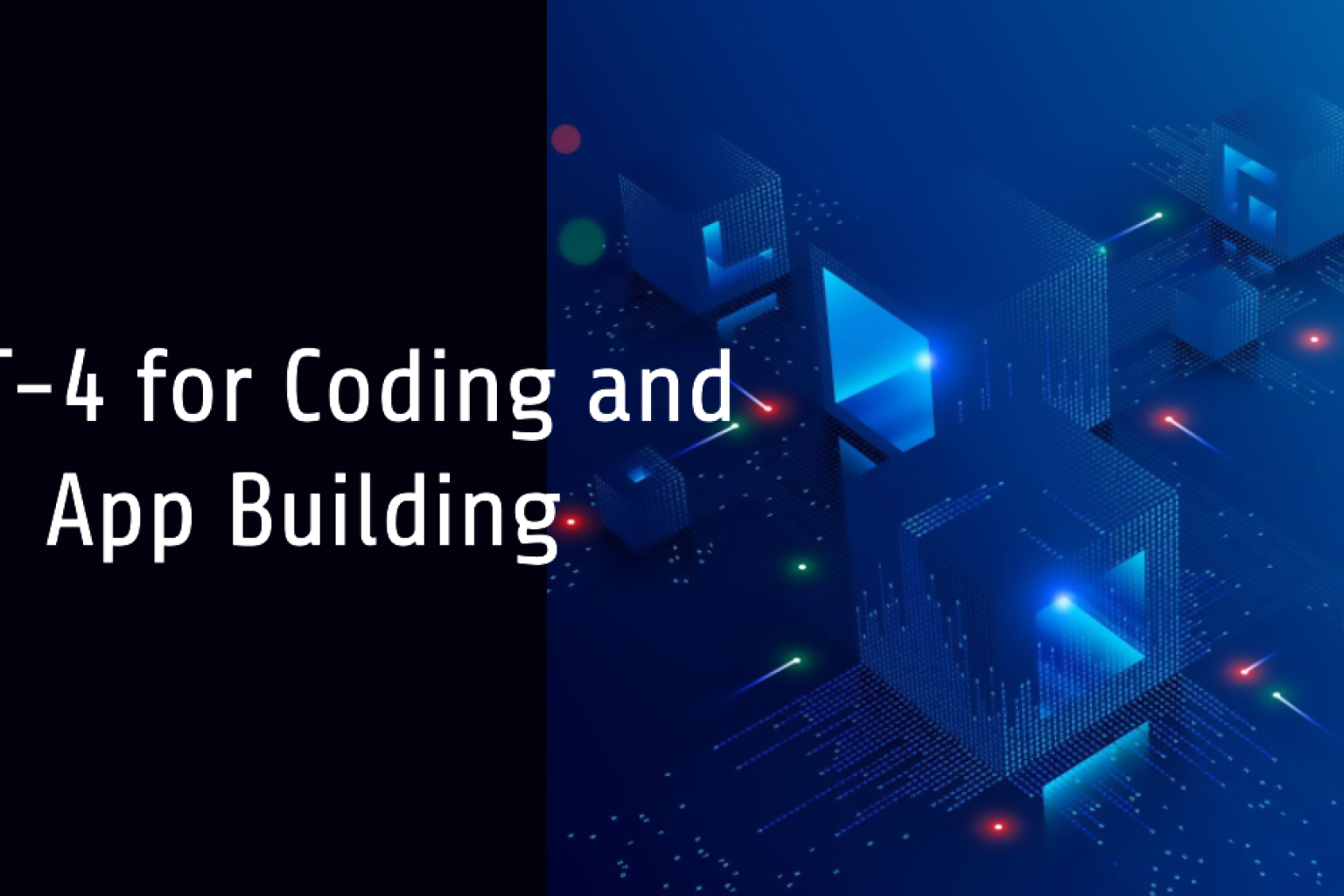
Artificial Intelligence (AI) has been revolutionizing various industries, and the recent release of GPT-4 has taken app development to new heights. With its advanced natural language processing capabilities, GPT-4 empowers developers to create AI-powered applications that can analyze, interpret, and respond to user inputs intelligently. This comprehensive guide will walk you through the process of leveraging GPT-4 to build cutting-edge applications. From generating ideas to designing, developing, and optimizing your app, you'll gain the knowledge and tools needed to harness the full potential of GPT-4 in your app development projects.
GPT-4 is an AI language model developed by OpenAI that boasts impressive capabilities, including the ability to process up to 8000 tokens of context in a single prompt. This opens up a world of possibilities for developers, enabling them to build intelligent applications that can understand and respond to user queries, provide personalized recommendations, and even generate human-like responses. The power of GPT-4 lies in its ability to leverage a vast amount of training data to understand and generate natural language, making it a valuable tool for app developers looking to create intuitive and engaging user experiences.
In this guide, we will explore the various aspects of GPT-4 app development, providing detailed steps, practical examples, and sample codes to help you grasp the concepts and implement them effectively. Whether you're a seasoned developer or just starting your app development journey, this guide will equip you with the knowledge and tools to leverage GPT-4 and take your app development skills to the next level.
Need to Quickly Create Charts/Data Visualizations? You can give VizGPT (opens in a new tab) a try, where you can use ChatGPT prompts to create any type of chart with No Code!
GPT 4 Coding Step 1: Generating Ideas with GPT-4
The first step in any app development project is to generate ideas. With GPT-4, you can harness the power of AI to generate innovative and unique ideas for your application. By providing GPT-4 with a prompt, such as "Give me 10 ideas for apps using the GPT-4 API," you can receive a list of creative suggestions that can serve as a foundation for your app concept. Let's explore the process of generating ideas using GPT-4:
-
Install OpenAI Python Package: Start by installing the OpenAI Python package, which provides the necessary tools to interact with GPT-4. You can install the package by running the following command in your terminal:
pip install openai
. -
Initialize GPT-4 API: Once the package is installed, you need to initialize the GPT-4 API. Obtain your API key from the OpenAI platform and set it as an environment variable in your development environment.
-
Create a Prompt: Craft a prompt that clearly specifies your request for app ideas using the GPT-4 API. For example, you can use the prompt: "Generate 10 unique app ideas that leverage the power of the GPT-4 API."
-
Generate Ideas: Utilize the
openai.Completion.create()
method to generate app ideas based on the prompt you created. Set themodel
parameter to "text-davinci-004" to leverage the GPT-4 model specifically. Retrieve the generated ideas from the
API response and store them for further evaluation.
import openai
openai.api_key = "YOUR_API_KEY"
def generate_app_ideas(prompt, num_ideas):
response = openai.Completion.create(
engine="text-davinci-004",
prompt=prompt,
max_tokens=50,
n = num_ideas,
stop=None,
temperature=0.7,
frequency_penalty=0.2,
presence_penalty=0.0
)
ideas = [choice['text'].strip() for choice in response.choices]
return ideas
prompt = "Generate 10 unique app ideas that leverage the power of the GPT-4 API"
num_ideas = 10
ideas = generate_app_ideas(prompt, num_ideas)
for i, idea in enumerate(ideas):
print(f"Idea {i+1}: {idea}")
By following these steps, you can tap into the creative potential of GPT-4 and generate a list of unique and innovative app ideas that can serve as a starting point for your development journey.
GPT-4 Coding 2: Designing the Application
Once you have a solid app idea in place, the next step is to design the user interface and overall structure of your application. Design plays a crucial role in creating a user-friendly and visually appealing app that will attract and engage users. In this segment, we will explore the steps to design your GPT-4-powered application:
-
Define User Flow: Start by defining the user flow of your application. Consider the main features and functionalities you want to include and how users will interact with them. Create a flowchart or wireframes to visualize the different screens and their relationships.
-
Choose a Design Tool: Select a design tool that suits your preferences and expertise. Popular options include Figma, Sketch, and Adobe XD. These tools provide a wide range of design elements and allow you to create interactive prototypes to test the usability of your app.
-
Create App Screens: Begin designing the individual screens of your application. Focus on creating a clean and intuitive interface that aligns with your app's purpose and target audience. Pay attention to typography, color schemes, and visual hierarchy to ensure a visually appealing and cohesive design.
-
Add GPT-4 Integration: Consider how GPT-4 will be integrated into your app's design. Determine the points where user inputs will be processed by GPT-4 and how the generated responses will be displayed to the user. Design the UI elements, such as input fields and chat interfaces, to facilitate seamless interaction with GPT-4.
from tkinter import *
from openai import GPT
# Initialize GPT-4
gpt = GPT(api_key="YOUR_API_KEY")
def generate_response(prompt):
response = gpt.get_completion(prompt)
return response['choices'][0]['text'].strip()
def submit_message():
user_input = input_field.get()
chat_text.insert(END, "You: " + user_input + "\n")
response = generate_response(user_input)
chat_text.insert(END, "GPT-4: " + response + "\n")
input_field.delete(0, END)
root = Tk()
root.title("GPT-4 Chatbot")
root.geometry("400x500")
chat_text = Text(root)
chat_text.pack()
input_field = Entry(root)
input_field.pack()
submit_button = Button(root, text="Send", command=submit_message)
submit_button.pack()
root.mainloop()
By following these steps, you can create a visually appealing and user-friendly design for your GPT-4-powered application. Remember to iterate on your design and gather feedback to ensure it aligns with your app's goals and user expectations.
GPT-4 Coding Step 3: Developing the Application
With the design in place, it's time to bring your GPT-4-powered application to life through development. In this segment, we will explore the steps to develop your application, including setting up the development environment, integrating GPT-4, and implementing the necessary functionalities:
-
Set Up the Development Environment: Begin by setting up your development environment. Install the necessary tools and frameworks for your chosen programming language. For example, if you're developing a web application, you may need to install Node.js, React, or Flask.
-
Integrate GPT-4 API: Connect your application to the GPT-4 API to leverage its powerful language processing capabilities. Use the OpenAI Python package to interact with the API and send prompts for text generation.
-
Implement User Input Handling: Create the functionality to capture user input and send it to the GPT-4 API for processing. This can be done through forms, chat interfaces, or other input mechanisms. Handle user input validation and ensure the data is formatted correctly for the API.
-
Process GPT-4 Responses: Receive the responses from the GPT-4 API and process them to extract the relevant information. Determine how to format and display the generated text to provide meaningful and coherent responses to user queries.
import openai
openai.api_key = "YOUR_API_KEY"
def generate_response(prompt):
response = openai.Completion.create(
engine="text-davinci-004",
prompt=prompt,
max_tokens=100,
temperature=0.7
)
return response.choices[0].text.strip()
@app.route("/chat", methods=["POST"])
def chat():
user_input = request.form.get("user_input")
response = generate_response(user_input)
return jsonify({"response": response})
By following these steps, you can develop the core functionalities of your GPT-4-powered application and enable users to interact with the AI-powered features seamlessly.
GPT-4 Coding Step 4: Optimizing the Application
Optimizing your GPT-4-powered application is essential to ensure optimal performance, user experience, and scalability. In this segment, we will explore various optimization techniques that can enhance the efficiency and effectiveness of your application:
-
Reduce Load Times: Long load times can negatively impact user experience. To mitigate this, consider implementing techniques such as lazy loading, caching, and minification of static assets. Additionally, optimize your server-side code and database queries to minimize response times.
-
Ensure Up-to-Date Information: GPT-4 relies on accurate and current data to generate meaningful responses. Regularly update your training data and leverage web scraping techniques to retrieve the most recent information from reliable sources. Consider implementing a scheduler to automate the data update process.
-
Implement Error Handling: Errors can occur during API calls or data processing. Implement robust error handling mechanisms to gracefully handle these errors and provide meaningful feedback to users. Monitor application logs and implement error tracking systems to identify and address any issues promptly.
-
Scale for Increased Traffic: As your application gains popularity, you may experience increased traffic. Ensure that your infrastructure can handle the load by implementing scaling strategies such as load balancing, auto-scaling, and vertical scaling. Monitor resource utilization and performance metrics to proactively address any scalability issues.
import time
from functools import wraps
def measure_execution_time(func):
@wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution time for {func.__name__}: {execution_time} seconds")
return result
return wrapper
@measure_execution_time
def process_user_input(user_input):
# Process user input and generate response
response = generate_response(user_input)
return response
By following these optimization techniques, you can improve the overall performance and user experience of your GPT-4-powered application, ensuring it can handle increased traffic and deliver up-to-date and accurate information.
GPT-4 Coding Step 5: Future Enhancements and Conclusion
With your GPT-4-powered application up and running, there are several future enhancements you can consider to further improve its functionality and user experience. In this segment, we will explore some ideas for future development and conclude our guide:
-
Enhance Natural Language Understanding: Continuously train and fine-tune your GPT-4 model to improve its natural language understanding capabilities. Consider incorporating user feedback and data from user interactions to enhance the accuracy and relevance of generated responses.
-
Implement Multi-Language Support: Extend the language capabilities of your application by integrating translation services or training GPT-4 on multilingual data. This will allow users from different language backgrounds to benefit from your app's AI-powered features.
-
Integrate Voice Input and Output: Explore the integration of voice input and output capabilities into your application. This can be achieved by leveraging speech recognition and text-to-speech APIs, enabling users to interact with your app through voice commands and receive audio responses.
-
Leverage User Analytics: Implement analytics and tracking mechanisms to gather insights on user interactions, preferences, and usage patterns. Utilize this data to further personalize the app experience, provide targeted recommendations, and improve overall user satisfaction.
Conclusion
In conclusion, GPT-4 has opened new doors for app development, allowing developers to leverage the power of AI and natural language processing. In this comprehensive guide, we have explored the process of creating a GPT-4-powered application, from generating ideas to designing, developing, and optimizing the app. By following the steps outlined in this guide and utilizing the sample codes provided, you can unlock the full potential of GPT-4 and create innovative, intelligent applications.
As technology continues to evolve, the possibilities for AI-powered applications are limitless. By staying updated with advancements in AI and integrating cutting-edge technologies into your app development process, you can create experiences that delight users and push the boundaries of innovation. So, embrace the power of GPT-4 and embark on your journey to revolutionize app development with AI.
Frequently Asked Questions (FAQ)
Is GPT-4 good for coding?
Yes, GPT-4 is a powerful tool for coding. It can assist developers by providing suggestions, generating code snippets, and offering insights on coding best practices. However, it's important to note that GPT-4 should be used as a complement to human coding skills, rather than a replacement. While GPT-4 can automate certain aspects of coding, it's still essential for developers to have a deep understanding of programming concepts and logic.
How to code with Chat GPT-4?
To code with Chat GPT-4, you can utilize the OpenAI Python package and interact with the GPT-4 API. The API allows you to send prompts or queries to GPT-4 and receive generated responses. By providing specific prompts related to coding challenges or questions, you can leverage GPT-4's language processing capabilities to get code suggestions, explanations, or insights. You can incorporate GPT-4 into your coding workflow by integrating it into your development environment or building a chat interface to interact with the AI model.
Can GPT-4 replace coders?
No, GPT-4 cannot replace coders. While GPT-4 is a powerful AI language model, it is designed to assist and augment the work of developers, not replace them. GPT-4 can automate certain aspects of coding, generate code snippets, and provide suggestions, but it lacks the critical thinking, creativity, and problem-solving abilities that human developers bring to the table. Coders play a crucial role in understanding complex requirements, designing robust solutions, and ensuring the quality and efficiency of the codebase.
What programming language is GPT-4?
GPT-4 is a language model and does not have a specific programming language. It is designed to process and generate natural language text. However, when interacting with GPT-4 through the API, you can use programming languages such as Python to send prompts and receive responses. The choice of programming language for integrating GPT-4 into your application depends on your preferences, the programming language support provided by the OpenAI API, and the requirements of your project.